Static Member Variables allow C++ Objects to share data. Unlike static member functions, the static member variables need extra efforts for understanding and implementation. The Sample Code provided in this post, describes how you can declare static member variable and use it across class objects. Unlike Global Variables, the static member variables are designed to be used by objects of C++ Classes only.
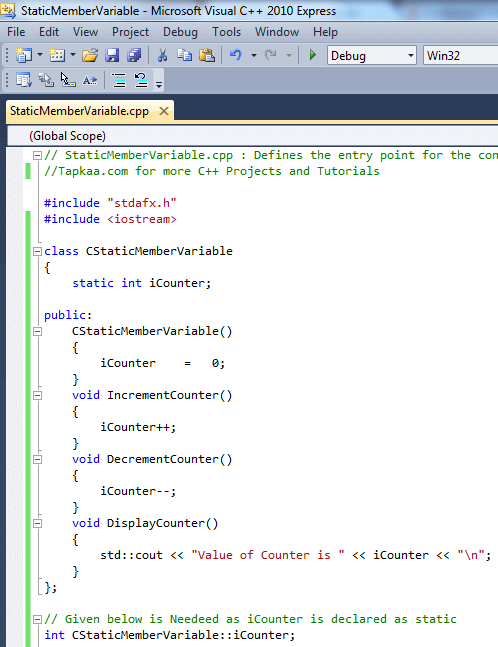
C++ Class with static Member Variable
The above screenshot displays a complete C++ class with a static member variable. Note there are extra functions defined to change the value of the static member variable and another function is defined to display the value of the static member variable. Although the functions are declared as public functions, however the member variable is declared as private so that only member functions of the call can read and modify the value of the static member variable.
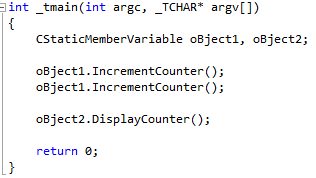
Using Class with Static member Variable
Now have a look at the above screenshot in which two different objects of the C++ class containing static member variable are created. Also note that only one object of the class id modifying the value of the static member variable of the C++ class and other object is used to display the value of the static member variable. Given below output of the above C++ Project will help you to understand and learn the behavior of the static member variables.
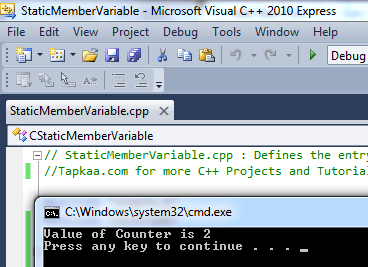
Output of C++ Sample Project Using Class with Static Member Variable
Note that even though the object displaying the value of the static member variable has not changed the value, even though it provides the modified member variable value. Static Member variables are singleton. In other words, the value of the static member variables are shared across all objects of the same C++ class. Given below sample code can save you time and efforts in typing the full source code of this sample C++ Project.
// StaticMemberVariable.cpp : Defines the entry point for the console application.//Tapkaa.com for more C++ Projects and Tutorials#include "stdafx.h"#include <iostream>class CStaticMemberVariable{static int iCounter;public:CStaticMemberVariable(){iCounter=0;}void IncrementCounter(){iCounter++;}void DecrementCounter(){iCounter--;}void DisplayCounter(){std::cout << "Value of Counter is " << iCounter << "n";}};// Given below is Needeed as iCounter is declared as staticint CStaticMemberVariable::iCounter;int _tmain(int argc, _TCHAR* argv[]){CStaticMemberVariable oBject1, oBject2;oBject1.IncrementCounter();oBject1.IncrementCounter();oBject2.DisplayCounter();return 0;}